반응형
[lv3/C++] 접근지정자
접근지정자는 클래스의 멤버 변수나 멤버 함수들의 접근 권한을 설정하는 키워드이다. 3가지(private, protected, public) 키워드가 있으며 각각 접근할 수 있는 범위가 다르다.
private
- 클래스 자신 및 친구(friend)라 선언한 클래스만 접근 가능
- private로 선언된 경우 자신의 멤버 함수 내부에서 멤버 변수들을 사용할 수 있다.
- friend로 선언된 "함수"나 "클래스"는 private로 선언된 변수나 함수를 접근할 수 있다.
protected
- 클래스 자신 및 파생 클래스(자식 클래스)만 접근 가능
- protected로 선언된 경우 자신의 멤버 함수 내부에서 멤버 변수들을 사용할 수 있다.
- 상속받은 자식클래스의 경우도 멤버 함수 내에서 접근 가능하다.
public
- main()함수를 포함한 모든 클래스가 접근 가능
#include <iostream>
using namespace std;
// 메인 클래스 (부모클래스)
class MainClass {
private :
int secret = 10;
protected :
int control = 20;
public :
int share = 30;
void GetMainClassValue() {
cout << "Private : " << secret << endl; // 접근 가능
cout << "Protected : " << control << endl; // 접근 가능
cout << "public : " << share << endl; // 접근 가능
}
friend class FriendClass; // 친구 선언
// friend void FriendFunction() // friend 선언시 외부 함수도 private에 접근 가능
};
// 친구 클래스
class FriendClass {
public :
void GetMainClassValue() {
MainClass mainClass;
cout << "Private : " << mainClass.secret << endl; // 접근 가능
cout << "Protected : " << mainClass.control << endl; // 접근 가능
cout << "public : " << mainClass.share << endl; // 접근 가능
}
};
// 자식 클래스
class ChildClass : public MainClass {
public :
void GetMainClassValue() {
MainClass mainClass;
cout << "Private : " << endl; // secret 접근 불가
cout << "Protected : " << control << endl; // 접근 가능
cout << "public : " << share << endl; // 접근 가능
}
};
int main()
{
cout << "Main Class" << endl;
MainClass mainClass;
mainClass.GetMainClassValue();
cout << "---------------------" << endl;
cout << "Friend Class" << endl;
FriendClass friendClass;
friendClass.GetMainClassValue();
cout << "---------------------" << endl;
cout << "child Class" << endl;
ChildClass childClass;
childClass.GetMainClassValue();
cout << "---------------------" << endl;
system("pause");
return 0;
}
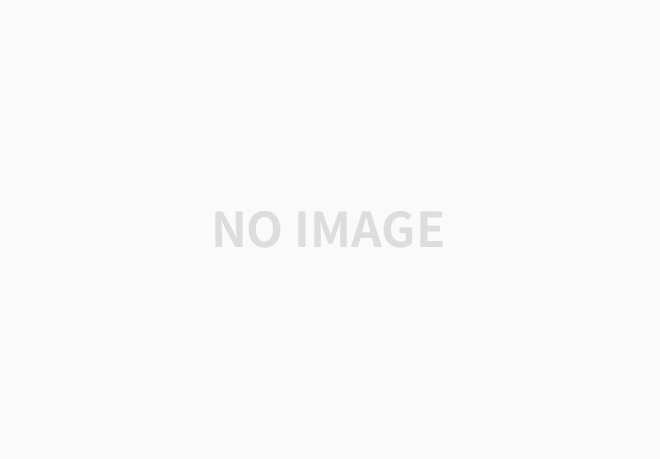
반응형
'Programming > C, C++' 카테고리의 다른 글
C++ 클래스 private접근, Friend 지정 사용 (0) | 2022.11.17 |
---|---|
C++ 오류 해결 Error:C4996, strcpy, strncpy 사용시 에러 발생 (0) | 2022.11.16 |
LV3 C++ 클래스와 객체 (0) | 2022.09.14 |
LV2 C++ 인라인(Inline) 함수 (0) | 2022.09.13 |
LV2 C++ 재귀(recursion) 호출 (0) | 2022.09.13 |